
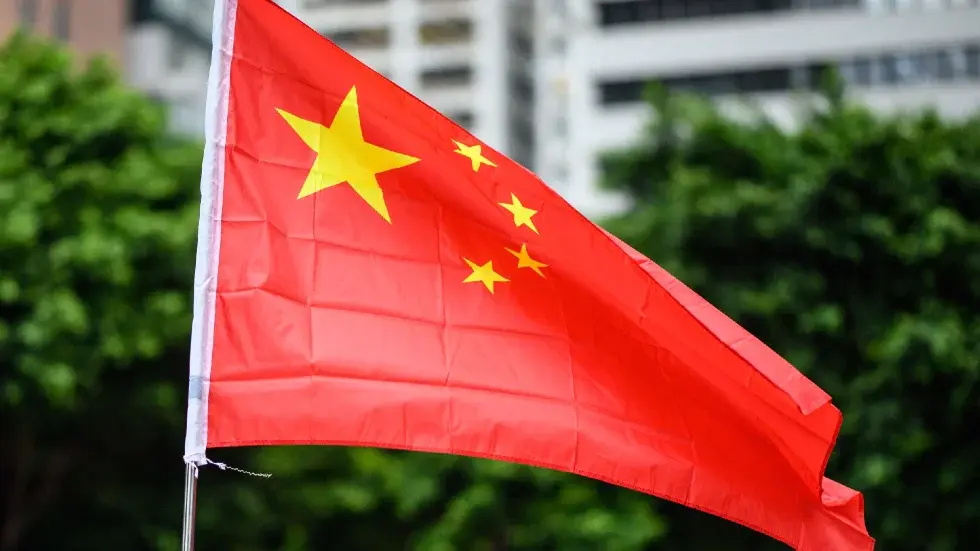
In the USA, while there is on paper separation of church and state, in practice there isn’t, because theists can control the state, and so they will just make up fake justifications for their religious laws they don’t believe in themselves. The only way to genuinely separate church and state is to not allow theists to control the state. Legally, the CPC controls the state, and you cannot join the CPC is you are a theist, so you will be limited in any sort of political ambitions.
Proselytizing is also illegal. If people come to your church it should because of their own personal interests or for family reasons because of your heritage. It shouldn’t be because some charlatan convinces you on the street or the television that you need to attend, because that just opens the way for a lot of exploitation, like snake oil salesmen and generally people who do harm to society at large.
If you are fine with those conditions, there are churches in China of many different faiths. The people who cry about persecution are often Christians who don’t like these conditions, they want to be able to control the state like in the US and they want to be able to proselytize and can’t. These are the evangelical types which definitely get persecuted in China but that’s a good thing.
Zionazis believe Israel has developed a kind of bomb that if you drop it on a house full of people, the blast will wrap itself around all Israelis leaving them unaffected. The explosion is able to check people’s citizenship status in real time to decide whether or not to incinerate them.